In this post I'd like to show you how to use JPA in NetBeans within a Java SE application. First of all you have to create a database and call it something like TestDB. I called my database 'Medienverwaltung' because I did a small JPA-tutorial from the new Javamagazin which had exactly the same database.
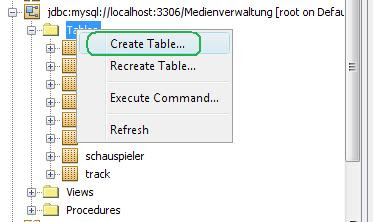
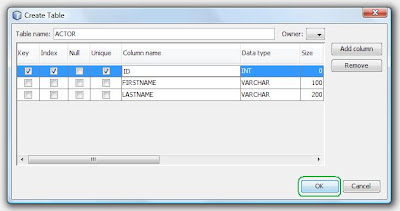
Create a very simple table without any references on other tables and call it 'ACTOR'. That's all you need for a first little JPA test. Now, create a JavaApplication in NetBeans. I called my application 'JPAGenTest'.
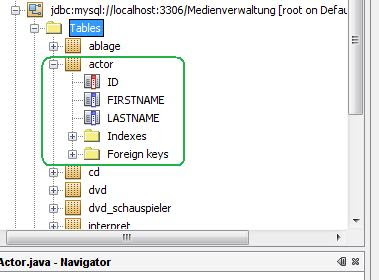
The next step is to generate an Actor-Entity-Class which will map the data into the corresponding 'ACTOR'-table in your database. A lot of errors will show up. That's because the necessary libraries are still missing.
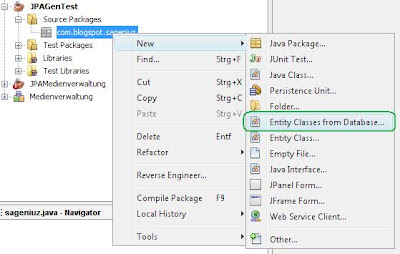
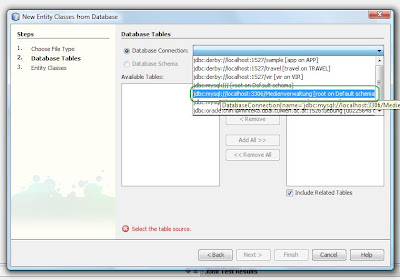
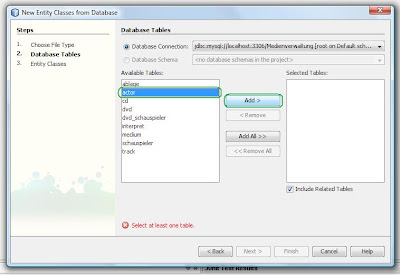
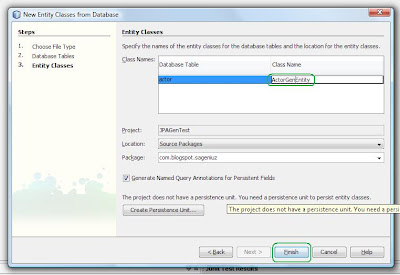
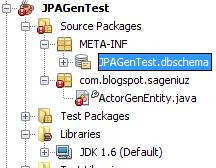
You can import them by configure your libriaries within the IDE or you create the persistent-unit and the libs will be imported automatically. You have to create this persistent unit anyway so I've decided to create it to import the missing libs.
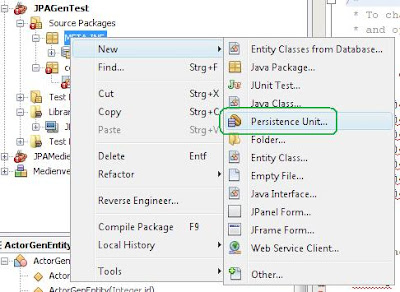
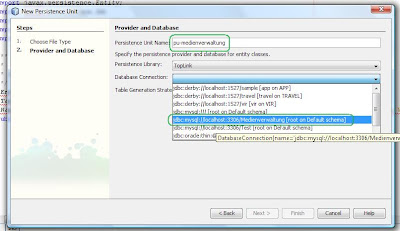
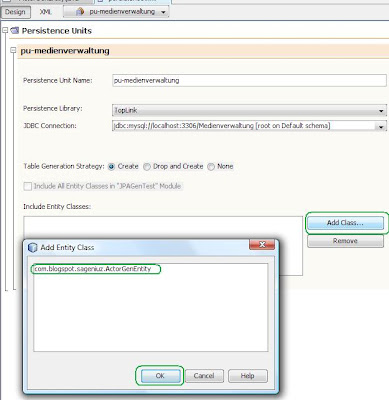
Now all errors are gone and you will be able to create more JPA functionality. The next step is to implement a Manager-Class which handles the JPA persistence-context. That means this class is responsible to provide CRUD functionality for your actor-instances and to communicate with your database.
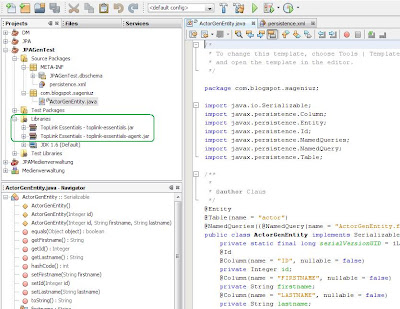
I've named this kind of class 'ActorManager' and implemented the following typically methods to interact with my database:
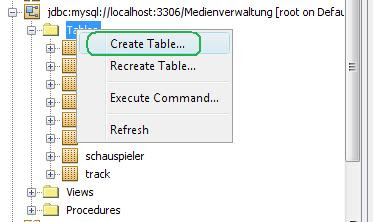
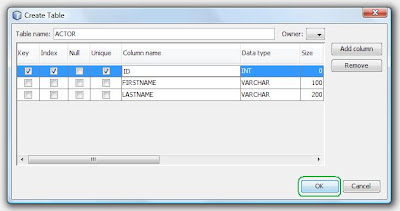
Create a very simple table without any references on other tables and call it 'ACTOR'. That's all you need for a first little JPA test. Now, create a JavaApplication in NetBeans. I called my application 'JPAGenTest'.
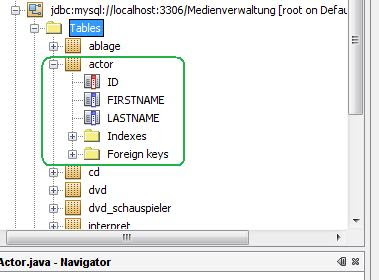
The next step is to generate an Actor-Entity-Class which will map the data into the corresponding 'ACTOR'-table in your database. A lot of errors will show up. That's because the necessary libraries are still missing.
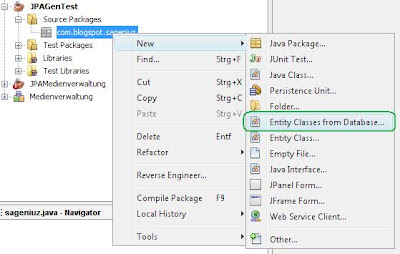
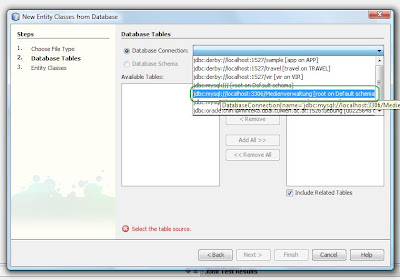
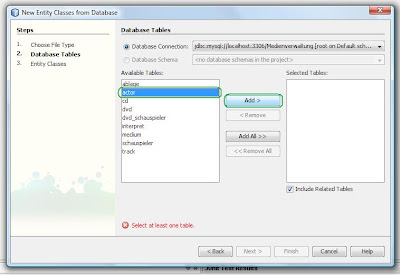
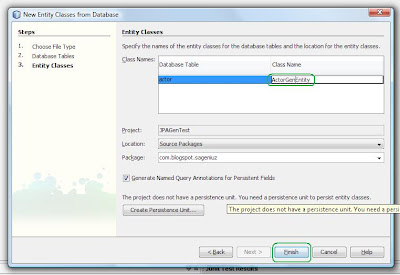
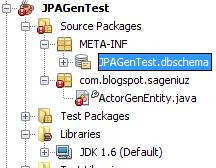
You can import them by configure your libriaries within the IDE or you create the persistent-unit and the libs will be imported automatically. You have to create this persistent unit anyway so I've decided to create it to import the missing libs.
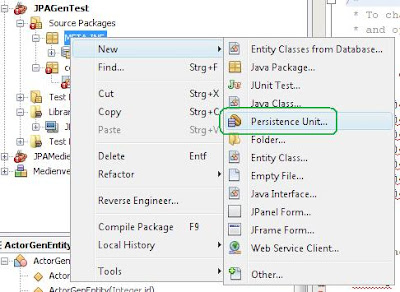
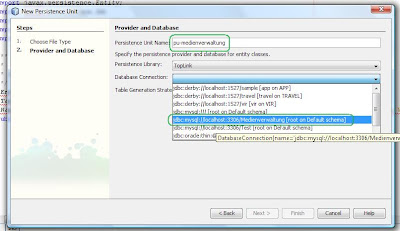
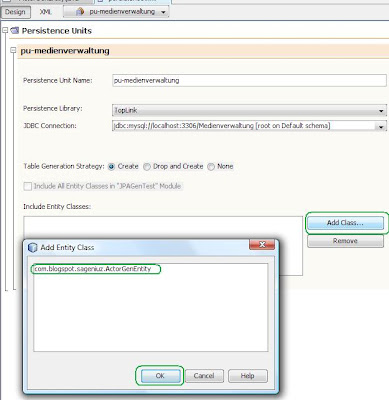
Now all errors are gone and you will be able to create more JPA functionality. The next step is to implement a Manager-Class which handles the JPA persistence-context. That means this class is responsible to provide CRUD functionality for your actor-instances and to communicate with your database.
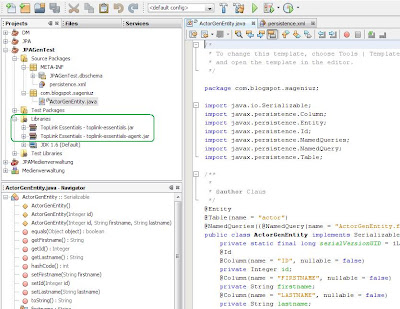
I've named this kind of class 'ActorManager' and implemented the following typically methods to interact with my database:
- createActor
- findById
- findByFirstname (NamedQuery)
- findByLastname (NamedQuery)
- updateActor
- removeActor
- getAll
- removeAll
NamedQuery is a JPA-Feature which was automatically created by NetBeans when you've created your Enitity-Class. It's a simple JPQL statement which usually will be used more often in your application.
Here is the code from the ActorManager:
package com.blogspot.sageniuz;
import java.util.Iterator;
import java.util.List;
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.Query;
/**
* Actor-Manager
* @author Claus Polanka
*/
public class ActorManager {
private EntityManager em;
public ActorManager(EntityManagerFactory emf) {
em = emf.createEntityManager();
}
public void createActor(ActorGenEntity actor) {
em.getTransaction().begin();
em.persist(actor);
em.getTransaction().commit();
}
public ActorGenEntity findById(Integer id) {
return em.find(ActorGenEntity.class, id);
}
public ListfindByFirstname(String firstname) {
Query query = em.createNamedQuery("ActorGenEntity.findByFirstname");
query.setParameter(firstname, em);
ListlistOfActors = query.getResultList();
return listOfActors;
}
public ListfindByLastname(String lastname) {
Query query = em.createNamedQuery("ActorGenEntity.findByLastname");
query.setParameter(lastname, em);
ListlistOfActors = query.getResultList();
return listOfActors;
}
public void updateActor(ActorGenEntity actor) {
em.getTransaction().begin();
em.merge(actor);
em.getTransaction().commit();
}
public void removeActor(ActorGenEntity actor) {
em.getTransaction().begin();
em.remove(actor);
em.getTransaction().commit();
}
public ListgetAll() {
Query query = em.createQuery("select a from ActorGenEntity a");
Listlist = query.getResultList();
return list;
}
public void removeAll() {
em.getTransaction().begin();
Query query = em.createQuery("select a from ActorGenEntity a");
Listlist = query.getResultList();
Iteratorit = list.iterator();
while (it.hasNext()) {
ActorGenEntity actor = it.next();
em.remove(actor);
}
em.getTransaction().commit();
}
public void close() {
em.close();
}
}
Testing the application is the next thing to do, so let's create a JUnit test-clase. Before you start implementing it check if you have already imported you database-driver or you will get an exception when executing your JUnit-test.
Here is the code for my test-class:
package com.blogspot.sageniuz;
import java.util.List;
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.Persistence;
import junit.framework.TestCase;
/**
* CRUD Test for Actor-Class.
* @author Claus Polanka
*/
public class TestActorManager extends TestCase {
private ActorManager am;
private EntityManager em;
private EntityManagerFactory emf;
private static final ActorGenEntity TESTACTOR1 = new ActorGenEntity(1, "Claus", "Polanka");
private static final ActorGenEntity TESTACTOR2 = new ActorGenEntity(2, "Barbara", "Ebinger");
private static final ActorGenEntity TESTACTOR3 = new ActorGenEntity(3, "Irene", "Polanka");
public TestActorManager(String testName) {
super(testName);
}
@Override
protected void setUp() throws Exception {
emf = Persistence.createEntityManagerFactory("pu-medienverwaltung");
em = emf.createEntityManager();
am = new ActorManager(emf);
}
@Override
protected void tearDown() throws Exception {
am.close();
em.close();
emf.close();
}
public void testCRUD() {
am.createActor(TESTACTOR1);
ActorGenEntity actor = am.findById(1);
assertEquals(actor.getFirstname(), "Claus");
actor.setFirstname("Barbara");
am.updateActor(actor);
actor = am.findById(1);
assertEquals(actor.getFirstname(), "Barbara");
am.createActor(TESTACTOR2);
am.createActor(TESTACTOR3);
Listlist = am.getAll();
assertEquals(list.size(), 3);
am.removeAll();
list = am.getAll();
assertEquals(list.size(), 0);
}
}
The following picture shows my project-tree and how your project-structure should also look like.
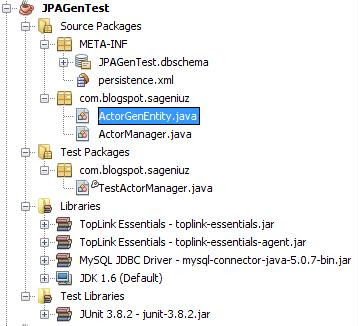
After running your test you should see something like the following.
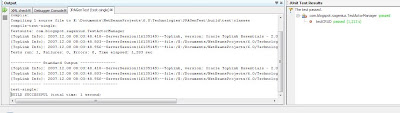
In the next days I will test more of the JPA features like support for derived Entities and stuff like that.
Cheers
202 comments:
«Oldest ‹Older 201 – 202 of 202Mau dapat uang tambahan KLIK DI SINI
Grate article. New to Addhunters? It's incredibly easy to become a member of our community, and FREE to list your classified ads to interact with all members. Every day, hundreds of listings get listed for free by our Addhunters members. You can always upgrade your membership and get ahead of the crowd. With Addhunters you're going to benefit from our international usages!Items listed on Add Hunters include electronics, pets, cars and, vehicles and other categories including land and property. The categories with the highest volume on the site are vehicles and property. For more details please visit our web site http://www.addhunters.com range rover qatar
Post a Comment